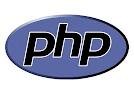 |
PHP: Hypertext Preprocessor,scripting language |
PHP File Upload
Example HTML code for file upload:
Form_page.php
upload_file.php [Simple Code]
Parameters of $_FILES
- $_FILES["file"]["name"] - the name of the uploaded file
- $_FILES["file"]["type"] - the type of the uploaded file
- $_FILES["file"]["size"] - the size in bytes of the uploaded file
- $_FILES["file"]["tmp_name"] - the name of the temporary copy of the file stored on the server
- $_FILES["file"]["error"] - the error code resulting from the file upload
upload_file.php [Code with Restriction on Upload, store the file in php temp folder]
upload_file.php [Code with Restriction on Upload, store the file in destination folder]
1 comment:
Excellent php tutorials.
Post a Comment